Introduction:
There are plenty of articles on the Pascal’s or Pingala’s triangle on the internet, so I wish not to revisit the explanation and the history about the triangle. Rather this blog post explains about one of the simplest way to produce or generate the triangle in Pythonic way. It talks about how one can generate the triangle using some of the Python concepts like, list comprehension, Python’s zip(), Formatting functionalities etc.
For more interesting facts on Pascals triangle. Please read the below web pages.
The Pascal Triangle and Pingala’s problem
Binomial Triangle Computer Binary System
The Coding:
To see the code, please visit my GitHub pages. The below section explains the code. The code starts with the python class which has two method, one is to collect the user input ‘get_level‘ and another is to generate the triangle ‘generate_pascal_traingle‘ along with a constructor which initializes the variables.
The constructor initializes the ‘row’ and ‘static_list’ which is list element with the value ‘0’ and ‘1’ respectively. It also initializes the level variable to ‘0’.
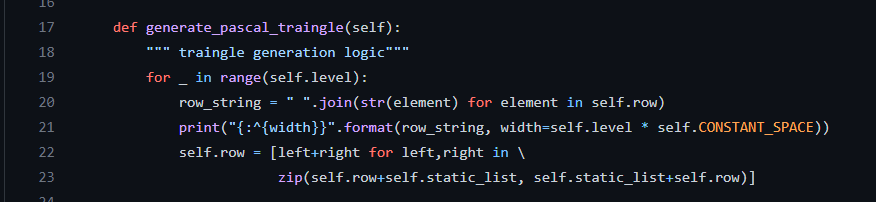
The ‘get_level’ is pretty simple method which collects the user input ‘level’ till where we need to generate the triangle. The ‘get_level’ method takes the user input and assigns it to the variable ‘level’
The ‘generate_pascal_traingle’ uses some of the Python concepts like, list comprehension, print formatting functions and zip() functionality. The method starts with the for loop which runs through the level mentioned by the user input. To understand the ‘_’ significance in the for loop, please visit the Quora page
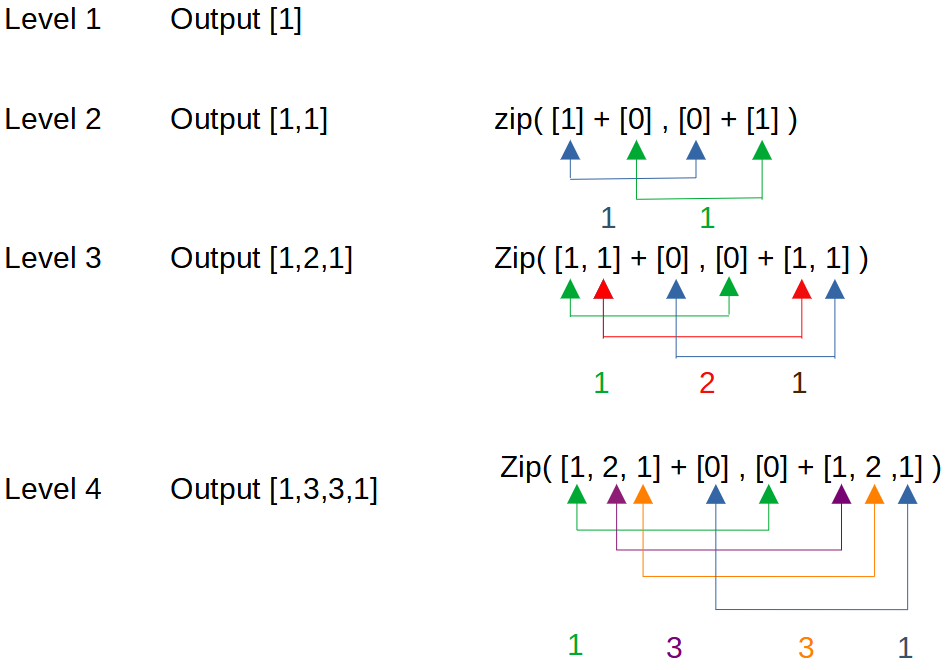
The line #20 generates the printing string, it uses string join and list comprehension functionality to generate the individual elements (numbers) from the genrated list and joins as single string with one white space.
The line #21 prints the string generated in the line # 16 with the Python formatting. The ‘width’ variable which is used in the formatting will sets the ‘width’ based on the user input ‘level’. I also used constant to increse the width by some more characters.
The line #22 will generate the each row for the triangle. Here we use the list comprehension and zip() functionality to generate the triangle row. The zip function takes the two list and generates the tuple by taking the individual elements from both the list. This is explained graphically in the below diagram.
After this, the for loop continues to print the generated row (line # 20) until the input level is reached.
This is one of the simplest way to generate the Pascal / Pingala triangle.
Happy Coding!